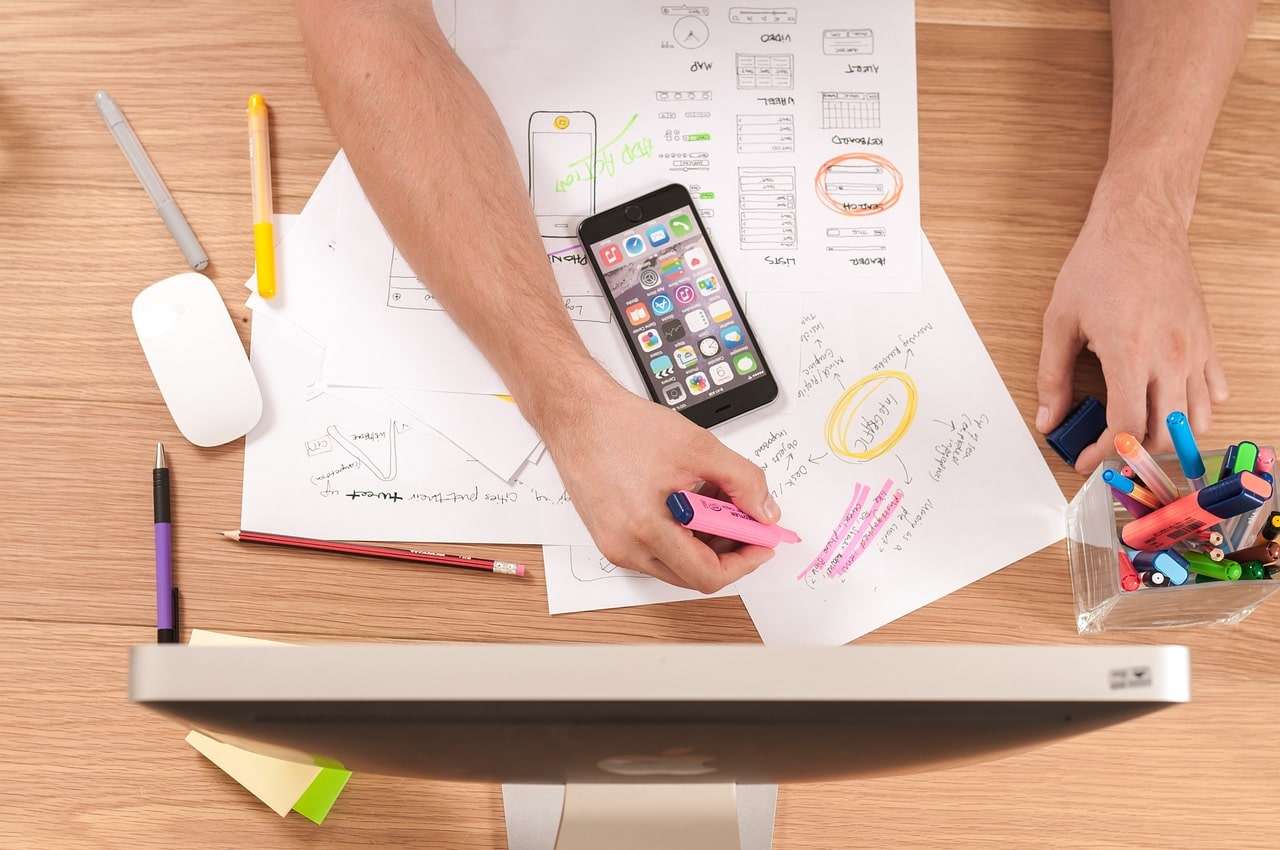
When new developers first attempt to tackle the beast that is Magento the overall size of the application and it's syntax can become a bit overwhelming. We've put together 5 really useful snippets that can be used in any Magento theme that should save new Magento developers quite a bit of time and headache when developing a Magento website.
1. Include A CMS Static Block In A Phtml Template
An easy way to introduce content into a Magento phtml template is by using a CMS Static Block, which can be created and managed in the admin dashboard at CMS > Static Blocks. CMS Static Block's are great because they can give the website owner an easy way to introduce text content, images, and code into predefined blocks in a template. Once you have a static block created, you can then call it in your template with the snippet below:
[php]
<?php echo $this->getLayout()->createBlock('cms/block')->setBlockId('static_block_id')->toHtml() ?>
[/php]
All you would need to do is swap "static_block_id" for the ID of the static block you'd like to use.
2. Get The Base Store URL In A Phtml Template To Create Links
When building links in your Magento phtml templates, relative URL's will simply not work and absolute URL path's are never suggested as they create pretty significant management issues when it comes to updating URLs. However, Magento makes it really easy to dynamically include your website's base URL in a php snippet so that you can easily build links to any page within your website without fear of URL updates (Remember to not include the trailing slash after your snippet as your website's base URL already includes it).
[php]
<a href="<?php echo Mage::getBaseUrl();?>path/to/your/link.html">Your Link Name Here</a>
[/php]
or
[php]
<a href="<?php echo Mage::getBaseUrl('path/to/your/link.html');?>">Your Link Name Here</a>
[/php]
3. Get The Base Skin URL In A Phtml Template
Often times, new Magento developers will want to include images in their phtml templates, but will attempt to include them as they would in a static HTML website, for instance a relative path to the image like 'assets/images/your-image-here.jpg'. In Magento, relative path's to images will not work and it's never good to include an absolute path in your templates because if the root domain were to change, you'd need to go back and manually update each one of those URLs. The correct way to do this in Magento would be to dynamically include the "Skin URL", or the direct URL to your templates directory with a PHP snippet. Using the same image path as mentioned before, this can be done in two ways (remember to not include the trailing slash after your snippet as your website's base URL already includes it):
[php]
<img src='<?php echo Mage::getBaseUrl(Mage_Core_Model_Store::URL_TYPE_SKIN); ?>assets/images/your-image-here.jpg'>
[/php]
or
[php]
<img src="<?php echo $this->getSkinUrl('assets/images/your-image-here.jpg'); ?>" >
[/php]
4. Get The Current Page URL In Your Phtml Template
Many times when developing a Magento website, you'll need to have access to the URL of the page you are currently on, for instance to save it in a variable for use in a conditional statement that will compare URLs with the intent on delivering custom content depending on the page the customer is viewing. This can be done with the snippet below:
[php]
<?php echo Mage::helper('core/url')->getCurrentUrl(); ?>
[/php]
Or if you'd like to store it in a variable:
[php]
<?php $current_url = Mage::helper('core/url')->getCurrentUrl(); ?>
[/php]
5.Check Whether A Customer Is Logged In Or Not
When creating the content for your Magento store, there may be pages, products, images, navigation links or basic text that you'd like to make visible only to users that are logged in. This snippet is always going to be used as part of a conditional 'if' statement, and can be used like this:
[php]
<?php if(Mage::getSingleton('customer/session')->isLoggedIn()): ?>
Your content for logged in users goes here....
<?php endif; ?>
[/php]
It can also be stored in a variable and used in conditional statements for nicer looking code:
[php]
<?php $logged_in = Mage::getSingleton('customer/session')->isLoggedIn();?>
[/php]