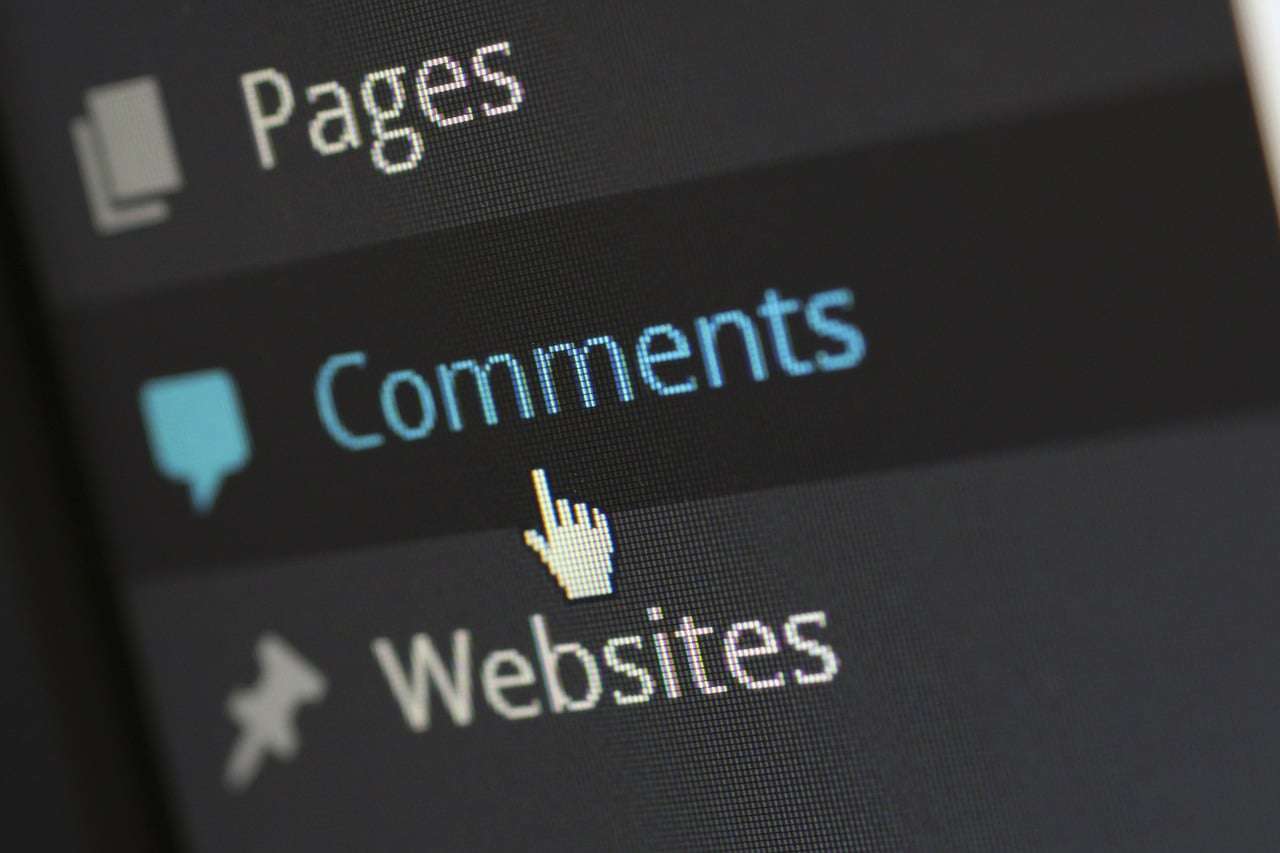
We posted an article last week with some very useful PHP snippets for Magento theming, so we figured it would be best to follow it up with another article that focuses on Wordpress theme creation.
One of the really great things about Wordpress is the number of easy to use PHP snippets that are available right out of the box. Keep in mind, when I say easy to use, that's really just my opinion that the PHP snippets created for Wordpress are easier to remember than those of Magento. For example, if you wanted to get the content of a given page in Magento, you would first need to get the page by ID and load the page, then you would call the page content with the snippet below:
[php]
<?php echo $page->getContent(); ?>
[/php]
However, within Wordpress, all you need to include is:
[php]
<?php echo the_content(); ?>
[/php]
So not only is the Wordpress snippet considerably shorter and easier to remember logically, but also requires a lot less 'pre-code' in order to access the page in question and it's contents. So without any further ado, here are 5 really useful PHP Snippets for Wordpress theme development.
1. Show A Custom Logo On The WP Login Page
A lot of times Wordpress developers will want to brand their Wordpress admin login page with either their logo or their clients logo. New developers will often try and find the code file or stylesheet that is adding the Wordpress logo in and then modifying it there, however this presents problems because the next time that Wordpress is updated, it will overwrite those changes that you had made. The best way to do this is to make the modifications through the functions.php file within your theme. By making the modification within your theme, your changes will be safe no matter how many times you update your Wordpress install. Here's how you would do that:
[php]
function custom_admin_logo() {
echo '<style type="text/css">
h1{ height:150px; }
h1 a { background-image:url('.get_bloginfo('template_directory').'/path/to/image.jpg) !important;}
.login h1 a { height:100%; }
</style>';
}
add_action('login_head', 'custom_admin_logo');
[/php]
2. Show The Featured Image From A Post or Page
One of the most usable features on any Wordpress page or post is the "Featured Image" box as it lets you attach an image to your content that can be accessed separately from the actual content. This makes creating posts and laying them out exactly as you'd like to with your CSS much easier to do. To include your featured image, you can just copy and paste the below snippet wherever you'd like it to show:
[php]
<?php
if ( has_post_thumbnail() ) { // First check to make sure that the featured image exists
the_post_thumbnail();
}
?>
[/php]
You can also pass the featured image size parameters, so in the case that your actual image is at 1800px X 1800px, but you only need it to load at 640px X 640px, you can pass it one of the following size parameters: 'thumbnail', 'medium', 'large', or 'full'. For example, if I wanted to show the image at medium size I would include it like this:
[php]
<?php
if ( has_post_thumbnail() ) { // First check to make sure that the featured image exists
the_post_thumbnail('medium');
}
?>
[/php]
3. Show or Hide Widgets Based on the Current Page
Another useful Wordpress trick that we will use on a lot of our Wordpress templates is hiding or showing different widgets based on the page that is being viewed. For instance, let's say we have a sidebar widget that allows for a user to book an appointment and that it is included on every page. Let's also assume that we also have a "Book An Appointment" page that someone can navigate to and book an appointment this way as well. In this situation, it would not really make sense to include the "Book An Appointment" sidebar widget on a page that already has the appointment scheduling form on it, so we will want to make sure that this widget will not be included on this page. To do that you can include the following in your functions.php file:
[php]
add_filter( 'widget_display_callback', 'hide_widget_appt', 10, 3 );
function hide_widget_appt( $instance, $widget, $args ) {
if ( $widget->id_base == 'appt_scheduler' ) { // change 'appt_scheduler' to widget name
if ( !is_page( 'appointments' ) ) { // change 'appointments' to the actual page name
return false;
}
}
}
[/php]
4. Exclude Certain Pages From Wordpress Search Results
One great feature that Wordpress makes available is the ability to create Custom Post Types to organize all sorts of different data. However, website owners do not always intend for the individual post types to be individually visible. For instance, let's say you've created a new custom post type called "FAQs" that will organize all of the "Frequently Asked Questions" your customers are typically looking for answers to. It's likely that you would use the custom post type to organize the questions, and then create a page with a loop of all of the posts from the FAQs to show all of the questions and answers on a page. However, in most cases, you would not want to make the individual question and answer post individually visible, as this would be very little content within the single page and largely unnecessary for your user experience. There are a number of ways to ensure that these posts are not indexed by search engines, but when using the native Wordpress search features, these posts will still be able to be found and navigated to. By using the snippet below, you can quickly filter out any posts that you do not want to be made searchable:
[php]
function filter_search($query) {
if ($query->is_search) {
$query->set('your_custom_post_type_here', 'post');
}
return $query;
}
add_filter('pre_get_posts', 'filter_search');
[/php]
5. Customize the Post Excerpt Length
Another great feature that Wordpress makes available in addition to 'the_content();', is 'the_excerpt();', which is of course an excerpt of the full content. As useful and usable as the excerpt snippet is, often times theme developers will want to modify the length of the snippet so that it will either take up more or less space in the design. There are actually two ways in which this can be done.
The first way to change the excerpt length is done through the functions file and will change the length of your excerpt globally, which means that any time you include the excerpt it will always be the same length. Assuming we want to shorten our excerpt to 35 characters, we would include the following in our functions.php file:
[php]
function custom_excerpt_length( $length ) {
return 40;
}
add_filter( 'excerpt_length', 'custom_excerpt_length', 999 );
[/php]
However, let's say you needed to include the excerpt in several areas throughout your theme, but you needed a different excerpt length depending on the location. Instead of modifying the excerpt globally, you could always modify it directly in the theme template by echoing it as a substring and trimming it down to whatever length you would like. You can do that with the snippet below:
[php]
<?php echo substr(get_the_excerpt(), 0,30); ?>
[/php]
If you have any trouble using any of the snippets above, or would like further clarification on their usage, feel free to drop in a comment below or email us directly.